This is a simple python script which uses the WebEx API. WebEx is a chat system created by Cisco. However its alot more then just that, its full on collaboration tool that can be used like Slack and Zoom at the same time. So it is more then just chatting, you can share screens as well.
What we do with this example is we simply use the API to write a message to the room (or specifically a small space). First we have the API get the room list, find the room by its name, then we get that rooms ID, then we send a message to that room ID.
Sidenote: If you have the room ID already you can shortcut a lot of the code
Sidenote: A room is any space (many people) or one on one conversation that you are having.
Getting API Key For Yourself for 12 hours:
More info on the API & developing with it is found here:
https://developer.webex.com/docs/api/getting-started
In fact, you need to go to that site to get the API Key. First login at the top left corner. Then scroll thru the guide and look up any guide. A good starting point is. API Reference -> Rooms . You should find the ability to copy your API Key into the clipboard directly from the page (make sure your browser is fully expanded on the screen).
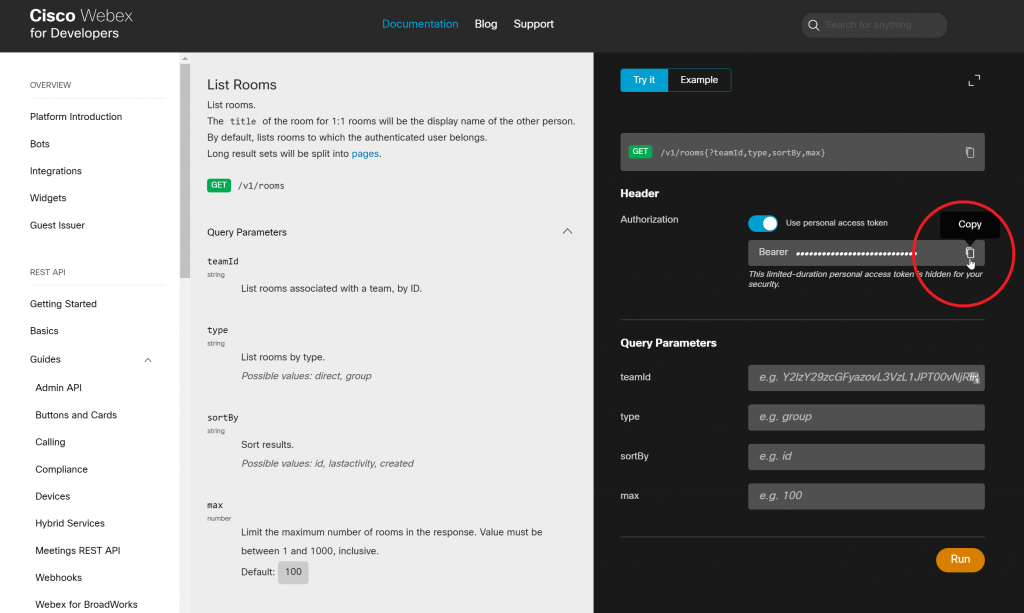
This will get a 12 hour API Key.
Getting A More Permanent API Key with a Bot:
To get a more permanent key (100 years) you have to create a WebEX API Bot / app (create bot here|more info on bots here). You give the bot a name, and a unique username, an icon (just like a user), and a description. The bot then gets a domain name tagged to it automatically with domain webex.bot – like an email username; so for bot username bot1, the full email username will be bot1@webex.bot. You can use this username to add your bot to your room. After submitting, you will get a Bot ID and Bot Token (You use the Bot Token as your API Key; its good for 100 years).
Sidenote: you can create bots, integrations, and guest issuers, and also login as yourself for 12 hours (explained above).
The Steps
- First open WebEx
- Create a room or space called “MyRoom-Test” (or whatever you want to call it)
- Make sure the user which API key is used is joined to the room.
- If you created an APP or Bot, make sure to join it to the room.
- Next, make sure you fulfill your python requirements, create the script and call the script with the correct environment variables in place.
The python script uses the requests python package which needs to be installed. Requests is an HTTP[s] package for python. Allows for easy manipulation of said protocol and therefore using REST API (which WebEx relies on)
I will not go in depth here on the code or REST API as there are plenty articles online, and this is mostly for my notes.
Sidenote: One thing, I didn’t do in this code is error handling.
Other Requirements:
- Python 3.9 as I use the f string format {var=} that was introduced then
- requests python package which can be installed with: pip install requests
Script (Find Room and Send Message):
import requests import os # run code by running: # export WBX_KEY="put key here" # python find-room-send-msg.py # The Message we want to send & room name TheMessage = "test message" WEBEX_ROOMNAME="MyRoom-Test" # setup vars WEBEX_API="https://api.ciscospark.com/v1/" # WEBEX_API="https://webexapis.com/v1/" WBX_KEY = os.environ.get('WBX_KEY', '') # setup session webex_session = requests.Session() webex_headers={"Authorization": "Bearer " + WBX_KEY} webex_session.headers.update(webex_headers) print(f"* {WEBEX_API=} {WEBEX_ROOMNAME=} {webex_headers=}") # get all my rooms webex_rooms = webex_session.get(url=WEBEX_API+"rooms") print(f"* {webex_rooms=}") # find my room - iterate thru rooms until we find out roomname and get that room id webex_rooms_json = webex_rooms.json() webex_room_id = None for i in webex_rooms_json["items"]: if i["title"] == WEBEX_ROOMNAME: print("- found room",i["title"],"its id is",i["id"]) webex_room_id = i["id"] # send message webex_message_json = { "roomId": webex_room_id, "text": TheMessage } webex_message = webex_session.post(url=WEBEX_API+"messages",json=webex_message_json) print(f"* {webex_message=}") print(f"* {webex_message.json()=}")
Note: that you can use the api.ciscospark.com and webexapis.com endpoint when calling the API, both will achieve same results.
How To Run It:
First set your WBX_KEY API Key environment variable. Replace PasteLongApiKeyHere with your actual key:
export WBX_KEY="PasteLongApiKeyHere" python find-room-send-msg.py
Example Output:
Personal information has been edited for security reasons.
me@host:~/src/webex-test$ python find-room-send-msg.py * WEBEX_API='https://api.ciscospark.com/v1/' WEBEX_ROOMNAME='MyRoom-Test' webex_headers={'Authorization': 'Bearer ZjY3NzEyZasdfF84_1eb65fdf-9643-417f-9974-ad72cae0e10f'} * webex_rooms=- found room MyRoom-Test its id is Y2lzY29zcasdfNDUzYjFmZDdjZGYy * webex_message= * webex_message.json()={'id': 'Y2lzY29zcGasdfLTg2YWMtOTdjOWE4NjIwYjhk', 'roomId': 'Y2lzY29zcasdfNDUzYjFmZDdjZGYy', 'roomType': 'group', 'text': 'test message', 'personId': 'Y2lzY29zcasdfC05MWNhMTRjMzE3YmQ', 'personEmail': 'bhbmaster@gmail.com', 'created': '2021-03-17T04:57:59.001Z'}
Also, you will see your “test message” in the actual WebEx application. If you used your API key it will come from you. If you used your Bots API key it will come from your bot.
The Script Assuming You Know The RoomID:
If you know the roomID you can make the code alot smaller obviously as we don’t have to find the roomID by title.
import requests import os # run code by running: # export WBX_KEY="put key here" # export WBX_ROOM_ID="put room id here" # python send-msg.py # The Message we send - roomname not needed, we get roomid from env vars below TheMessage = "test message" # setup vars - we get api key and roomid from environment variables WEBEX_API="https://api.ciscospark.com/v1/" # WEBEX_API="https://webexapis.com/v1/" WBX_KEY = os.environ.get('WBX_KEY', '') WBX_ROOM_ID = os.environ.get('WBX_ROOM_ID', '') # setup session webex_session = requests.Session() webex_headers={"Authorization": "Bearer " + WBX_KEY} webex_session.headers.update(webex_headers) print(f"* {WEBEX_API=} {WEBEX_ROOMNAME=} {WBX_ROOM_ID=} {webex_headers=}") # send message webex_message_json = { "roomId": WBX_ROOM_ID, "text": TheMessage } webex_message = webex_session.post(url=WEBEX_API+"messages",json=webex_message_json) print(f"* {webex_message=}") print(f"* {webex_message.json()=}")
Then you would call this code like this:
First set your WBX_KEY api key environment variable by replacing PasteLongApiKeyHere with your actual key. Do the same but with the long Room ID for PasteLongRoomIDHere.
export WBX_KEY="PasteLongApiKeyHere" export WBX_ROOM_ID="PasteLongRoomIDHere" python send-msg.py
The output will be similar to the previous section but shorter as we don’t have to find the room and you will get a text message in your WebEx as expected.
The end,